Most people know what Enums is, where we need it, and where we can use it.
Berif Summary
Enum is a special class, that we can use to represent constant values or fixed values.
Syntax of Enums:-
enum <Name of Enum>{
value1,
value2,
value3
}
Example:-
enum TypeOfMedia{
image,
video,
mp3
}
If we want to show some text and extensions of these media types we need to create separate methods to return specific values of specific enums.
Take an example:-
Need to show the text Value of these enums, we have to create functions and return the value of it.
static String getMediaName(TypeOfMedia typeOfMedia){
if(typeOfMedia == TypeOfMedia.image){
return 'Image';
} else if(typeOfMedia == TypeOfMedia.video) {
return 'Video';
} else if(typeOfMedia == TypeOfMedia.mp3){
return 'Audio';
}
return 'NA';
}
The above example will return the Name of the media type with a specific Enum. Like Image, Video, and Audio.
Sames goes for extension, I have to create separate methods to get extensions of specific Media types, as below code:-
static String getExtensionOfMediatype(TypeOfMedia typeOfMedia){
if(typeOfMedia == TypeOfMedia.image){
return 'JPEG, PNG, GIF';
} else if(typeOfMedia == TypeOfMedia.video) {
return 'MP4, MOV, AVI';
} else if(typeOfMedia == TypeOfMedia.mp3){
return 'MP3, WAV, FLAC';
}
return 'NA';
}
There are lots of chances we can forget to enter values in these methods while entering a new enum in TypeOfMedia.
New Superpower Unlock in Enum | Enhanced Enums
Dart allows us to create new fields and methods in the enum class.
To declare enhanced Enum, Need to know similar syntax with some extra information:-
- All variables must be final.
- All constructors must be constant.
- The variables cannot be declared in the enum.
- All enums must be declared at the beginning of the enum class and there must be 1 variable declaration in enums.
Now we are going to make Enhanced Enum in Dart.
We will go with the above example:-
enum TypeOfMedia {
/// Declaration of enums here
image(
name: 'Image', /// Pass the Name of image enum
extensions: 'JPEG, PNG, GIF', /// Pass the extension related to Image
),
video(
name: 'Video',
extensions: 'MP4, MOV, AVI',
),
mp3(
name: 'Audio',
extensions: 'MP3, WAV, FLAC',
);
/// Make sure end of enum type need make separation for that we use ";" here
/// Constructor created with const
const TypeOfMedia({
required this.name,
required this.extensions,
});
final String name;
/// variable declared with final
final String extensions;
/// variable declared with final
}
How can I use these values in code?
You guys can use it in very easy ways. Wait I will show you with example;
void main(){
/// Here TypeofMedia is Enum class name
/// mp3 is enum type
/// name is variable we used for check name of type
print(TypeOfMedia.mp3.name);
/// Here TypeofMedia is Enum class name
/// mp3 is enum type
/// extension is variable we used for check extension I entered in class
print(TypeOfMedia.mp3.extensions);
}
Output
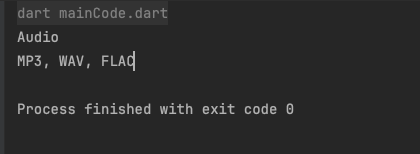
As we can see in the above output, in mp3 we gave a value of the name “Audio” and in the extension, we gave “MP3, WAV, FLAC”.
Using these Enhanced Enums we don’t need to create separate methods to get values and there is no chance of error to set values in the method.
I hope this will help you a little bit to use Enums efficiently in Dart.
Thanks & Regards
Namaste 🙏 from Shivam Sharma